JavaScript - Syntax
JavaScript Tutorial 02
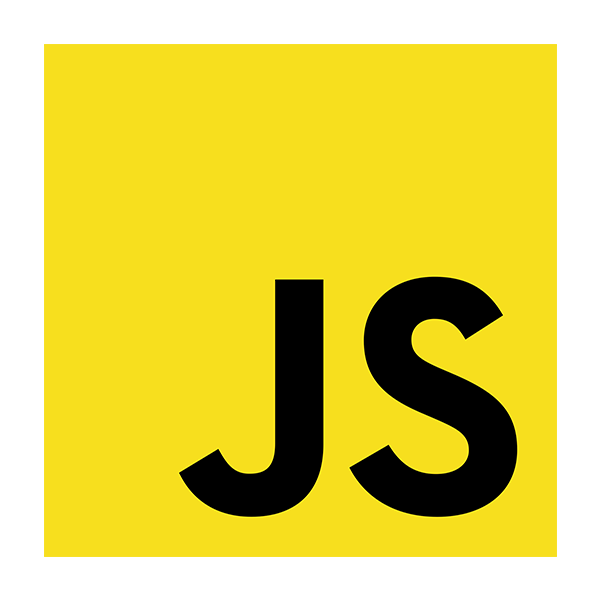
We covered the introduction, history, and overview of JavaScript(JS) in our initial JavaScript tutorial. If you haven’t read it, we thoroughly encourage you to go ahead and read JavaScript Tutorial 01 before reading this. That way, you won’t miss any important point about JavaScript.
In this tutorial, you will learn about JavaScript syntax. JavaScript syntax is the set of rules used to create JavaScript applications.
JavaScript can be implemented using JavaScript statements that are placed within the <script> </script> tags in a web page. The <script> tags, which contain the JS code, can be placed anywhere on your HTML page. A web page may contain multiple <script> tags between the <head> or <body> tags. However, it is generally recommended to keep <script> tags within <head> tags.
The browser executes all the script tags, starting from the first script tag from the beginning. Before loading and rendering the components of the <body> tag, the browser loads all scripts included in the <head> tag.
Therefore, if JavaScript files or code will be used to generate the user interface, they must be included in the <head>. Before the closing </body> tag, all additional scripts must be positioned. This will speed up the loading of the page.
As we discussed in our JavaScript tutorial 01, the <script> tag can also be used to include an external script file to an HTML web page by using the src attribute.
The following is a simple example of JavaScript syntax.
<script>
JavaScript code
</script>
The <script> tag has two important attributes. Those are,
- Language − The scripting language that you are using is defined by this attribute. Usually, javascript will be its value.
- Type − This attribute indicates the scripting language that is being used and its value must be set to “text/javascript”.
This is how your JavaScript segment will appear with those two attributes.
<script language = "javascript" type = "text/javascript">
JavaScript code
</script>
Case Sensitive
JavaScript is a case-sensitive scripting language. This necessitates appropriate letter capitalization is required when typing keywords, variables, function names, and any other identifiers. For instance, Name is not equal to nAme, myobject and MyObject are different, etc.
Identifiers
Identifiers are simply names used for variables, parameters, functions, classes, etc. An identifier name can start with,
- Letter (a-z, or A-Z)
- An underscore(_)
- Dollar sign ($)
Keywords & Reserved words
You can use whatever the name for your identifiers except for the reserved keywords. JavaScript has a list of reserved keywords that have specific uses. So you cannot use those keywords as identifiers by rule.
Some of the reserved keyword on JavaScript: function, switch, while, return, try, catch, in, void, class
This is the foremost tutorial in the JavaScript programming language tutorial series.suntechit.com.au
JavaScript Variables
Variables are used in programming languages to store data values. To declare variables in JavaScript, you must use the var, let, or const keywords, values are assigned to variables using the equal sign.
In the below example, the “name” is defined as a variable.
<script>
var name;
name = "Dave";
</script>
There are two types of variables in JavaScript.
Those are,
- Local variables: Declare a variable inside of a function.
- Global variables: Declare a variable outside of a function.
Whitespace
Whitespaces are ignored by JavaScript. Characters that separate other characters from each other are referred to as whitespace. However, whitespace can be used to format the code, making it simpler to read and maintain. It should be noted that JavaScript bundlers eliminate all whitespaces from JavaScript files and combine them into a single file before deployment. JavaScript bundlers accomplish this in order to make the JavaScript code shorter and quicker to load in web browsers.
Semicolons
Semicolons (;) are used to separate JavaScript statements. And it is a good programming practice to use semicolons.
However, if you want to avoid the semicolon, then you need to type JS statements on separate lines as shown below example,
<script language = "javascript" type = "text/javascript">
<! -
var x= 2
var z= 5 -->
</script>
But if you want to type your JS statements on a single line as follows, you must use semicolons.
<script language = "javascript" type = "text/javascript">
<! -
var x= 2; var z= 5;
-->
</script>
Comments
Code written after double slashes // or between /* and */ is treated as a comments. By using comments you can add notes on your JavaScript code. But don’t worry, JavaScript will ignore those comments when executing the code.
JS supports two types of comments as single-line and block comments.
Single-line comments
If you add double slashes(//) before typing the code, all the text following the // on the same line turns into a comment.
For example:
//this is a single line comment
Block comments
Block comment begins with a forward slash and asterisk /* and ends with the opposite */ as shown in the following example:
/* This is a block comment
that can span multiple lines */
Statements
Statements declare variables or instruct the JavaScript program to do a task. A simple statement is terminated by a semicolon (;) (semicolon is optional).
According to the following example, JS declares a variable and shows it to the console:
let message = "Welcome to JavaScript tutorial 02";
console.log(message);
Blocks
A block is a sequence of zero or more simple statements. A block is delimited by a pair of curly brackets {}.
if (window.localStorage) {
console.log('The local storage is supported');
}
Number
JavaScript allows us to work with any type of number such as integer, float, etc. Keep in mind that the number must NOT be written between quotation marks (“”). If you write a number between quotation marks (“”), then the number simply turns into a string.
JavaScript operators
JavaScript operators are used to calculate values.
- Arithmetic operators ( +, -, *, / ) — used to compute the values
- Assignment operator ( =, +=, %= ) — used to assign the values to variables.
An example where you can use JS operators:
<script>
var a, b, sum, sub, multiply, divide; //declare the variables
// Assign value to the variables
a = 10;
b = 2;
// to add two numbers you must use (+) sign
sum = a + b; //output is 12
// to add two numbers you must use (-) sign
sub = a - b; //output is 8
// to multiply two numbers you must use (*) sign
multiply = a * b; //output is 20
// to divide two numbers you must use (/) sign
divide = a / b; //output is 5
document.write(sum);
document.write(sub);
document.write(multiply);
document.write(divide);
</script>
Expressions
Expression is the combination of values, operators, and variables. It is used to compute the values.
For example:
<script>
var x, num, sum;
x = 10;
y = 20;
sum = x + y; //output is 30
num = x * y; //output is 200
document.write(num + "<br>" + sum); //use <br> to give line break
/* output is,
200
30
*/
</script>
You can read more case studies on https://suntechit.com.au/
Comments
Post a Comment